1. class
class variables을 먼저 선언, 그 뒤에 클래스 메소드들을 선언한다.
cout은 출력과 같은 기능이다. 변수 출력 시, 변수 이름을 <<사이에 적고, 문자열 또한 그렇다.
줄 띄움 기능은 따옴표로 둘러싸지않고 endl을 적는다.
1
2
3
4
5
6
7
8
9
10
11
12
|
class Message {
private:
string name = "Omar";
int age = 22;
float marks = 95.65;
public:
void display() {
cout << name << " is " << age << " year Old: " << endl;
cout << "He got " << marks << "% marks in his exam: ";
}
};
|
2. object
1
2
3
4
5
6
|
main() {
Message msg;
msg.display();
return 0;
}
|
객체 생성과 함수 접근은 자바와 비슷하다.
3. Abstraction (추상화)
외부에 필요한 정보만을 제공한다.
예를 들어, 우리가 폰을 사용할 때 우리는 어떻게 폰이 작동하는지 모른다.
이것과 같이 우리가 printf()를 사용할 때, 내부 동작을 모르고도 사용한다.
- 추상화의 이점 : 내부 상세정보들을 보호할 수 있다 (정보 은닉)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
#include<iostream>
using namespace std;
class hide {
private: //private member -> abstraction
int x, y;
public:
hide() { //constructor
x = 10;
y = 20;
}
void show() {
cout << " The value of x is: " << x << endl;
cout << " The value of y is: " << y;
}
};
main() {
hide obj;
return 0;
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4f; text-decoration:none">Colored by Color Scripter
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none">cs |
변수 x와 y를 private로 선언함으로서, 추상화하였다.
이런 정보들은 getter 및 setter 메소드를 통해 접근해야 한다.
4. Encapsulation
Data Encapsulation은 데이터를 묶고, 그것들을 사용하는 함수들을 묶는 매커니즘이다.
즉 하나의 엔티티로 통합을 하여, 다른 세부 사항들을 숨기는 것이다.
Data Abstraction은 사용자로부터 인터페이스만 노출시키고, 중요한 정보들은 숨기는 매커니즘이다.
Encapsulation의 코드적 예로는, 코드적으로 어떤 연산이 수행되던지 result만 출력하는 등이 있다
5. Inheritance
한 클래스에서 다른 클래스로 '데이터와 함수'를 얻어간다.
- 이점 : 시간과 메모리를 아끼며, 더 적은 저장공간이 요구된다

문법은 위 사진과 같다.
자바의 extends와 유사하게, 자식 클래스를 먼저 적고, 콜론 다음에 상속받을 부모 클래스를 적는다.
부모 클래스 앞에는 접근자를 명시해준다.
ex) class Triangle : public TwoD_Shape
6. Polymorphism (다형성)
사전적 의미는 '여러 모양'이다.
한 가지 형태로 여러가지의 기능을 수행할 수 있는 것을 의미한다.
다형성의 시작은 상속에서부터 시작하는데, 부모 클래스가 가지고 있는 메소드는 자식 클래스도 가지게 된다.
하지만 자식 클래스는 거기에 추가하여 다른 클래스도 가질 수 있다.
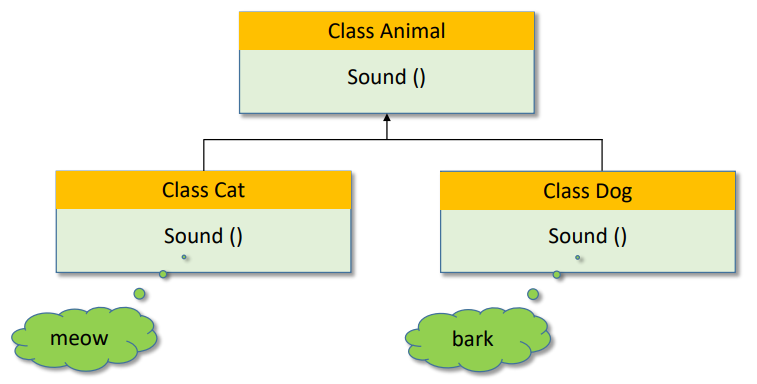
두가지 polymorphism 형태가 있는데,
- compile time polymorphism : method overloading, mothod overriding
- run-time polymorphism : using virtual function
<mothod overloading>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
#include<iostream>
using namespace std;
class math {
public:
void result(int x, int y) {
cout << " The result is : " << x + y;
}
void result(int x, int y, int z) { //method overloading with extra parameter
cout << " The result is : " << x + y + z;
}
};
main() {
math obj;
cout << endl;
return 0;
}
http://colorscripter.com/info#e" target="_blank" style="color:#4f4f4f; text-decoration:none">Colored by Color Scripter
http://colorscripter.com/info#e" target="_blank" style="text-decoration:none">cs |

위 코드를 보면, 같은 result라는 이름의 함수이지만, 파라미터의 수가 다르다.
<method overriding>
1
2
3
4
5
6
7
8
9
10
11
12
13
|
class base {
public:
void output() {
cout << " Its Base Class: " << endl;
}
};
class derived : public base {
public:
void output() {
cout << " Its Derived Class: " << endl;
}
};
cs
|
자식 클래스인 derived는 부모 클래스 base를 상속받는다.
부모 클래스에 있는 output()을 overriding해서 derived에서 다시 작성하였다.
'OOP' 카테고리의 다른 글
TermProject [1] (0) | 2019.11.12 |
---|---|
c++ [ 2 : Hello, World! ] (0) | 2019.10.16 |